Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts

Harnessing the Power of In-Memory Caching in Go
In the realm of software development, performance optimization is a constant priority, especially for applications that demand high throughput and low latency. One effective way to achieve this is through the use of in-memory caching.
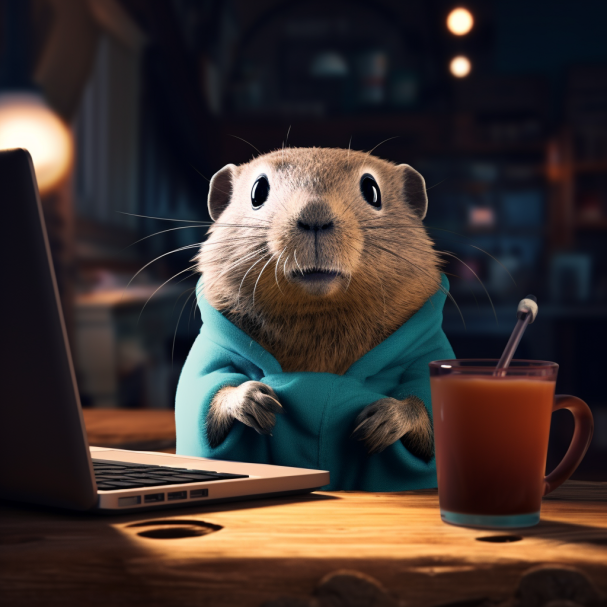
Unlocking Sophisticated Capabilities with Go Struct Tags
In the realm of Go programming, struct tags are powerful yet often underutilized tools that can dramatically enhance the functionality of your applications. These small pieces of metadata attached to struct fields allow for rich configuration and integration with other systems, making them essential for tasks such as data serialization, validation, and database interaction.

Implementing State Machine Patterns in Go
State machines are a fundamental design pattern in software engineering, used to manage complex states within applications. In Go, implementing a state machine can be both efficient and straightforward due to the language’s simplicity and robust features. In this blog post, we’ll explore how to design and implement a state machine in Go, leveraging its strong typing, interface, and concurrency features to create a clean and scalable solution.

Mastering Python: How to Use the 'or' Operator for Efficient Default Assignments
In the world of Python programming, the principle of writing concise and readable code is often highlighted by the term "Pythonic". This term refers to code snippets that not only follow Python syntax but also embrace Python's philosophy of simplicity and directness. One such example of Pythonic code is the use of the or operator in assignments, such as name = name_input or 'None'.
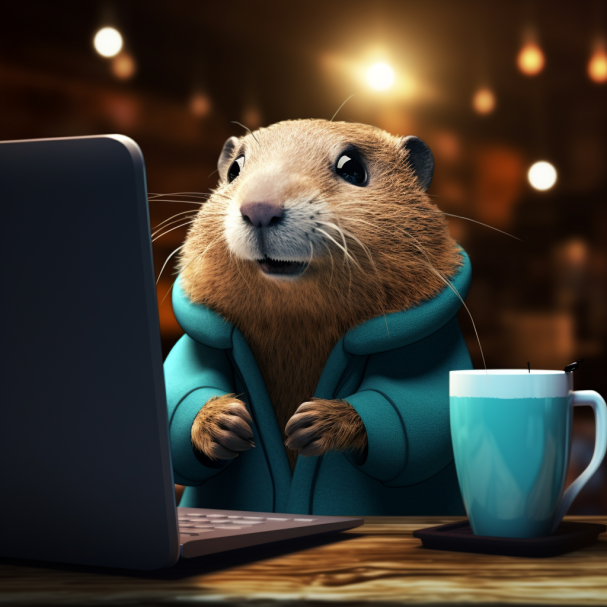
Leveraging Interface Checks in Go: Ensuring Type Safety
In this blog post, we delve into the importance of interface checks in Go programming. Using the example var _ App = (*Application)(nil), we demonstrate how to enforce type conformity to interfaces at compile time, thereby preventing potential runtime errors. Through a practical example, we illustrate how this technique serves not only as a safeguard but also as clear documentation for developers. Learn how to enhance your Go code's reliability and maintainability with interface checks.
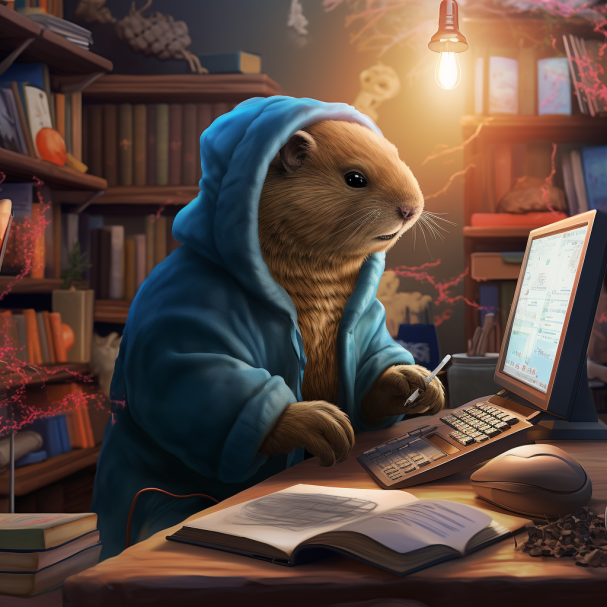
Pointer vs. Value Receivers in Go: Maximizing Efficiency in Your Code
Learn about the fundamental distinctions between pointer and value receivers in Go programming, and how these affect memory management, method performance, and code organization.

Avoiding Resource Leaks: Safe File Handling with Readers and Writers in Go
Understanding the use of readers and writers in Go can greatly enhance your coding efficiency and capability. This blog post dives deep into how you can leverage these tools with practical code examples.
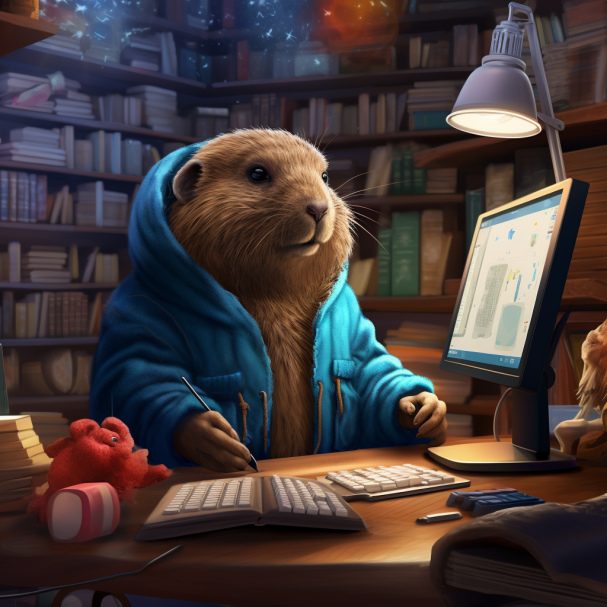
Boosting API Efficiency with Request Coalescing in Go: A Developer’s Guide
Request coalescing is a powerful strategy in Golang programming that optimizes API efficiency by reducing redundant server calls. Discover how it works and how to implement it effectively.
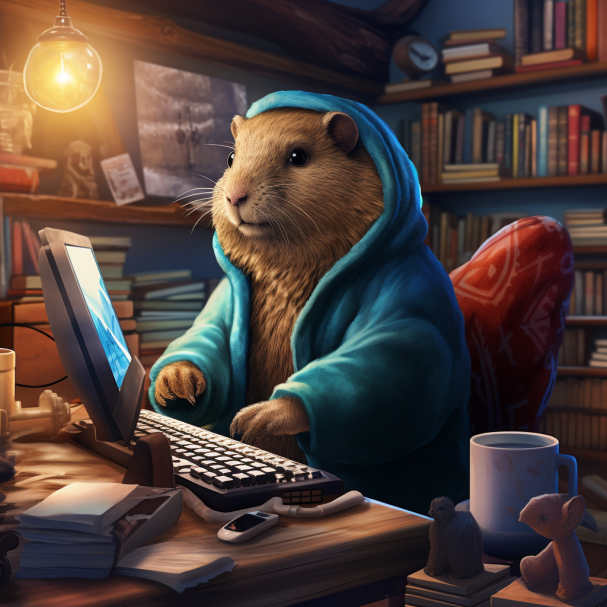
Advanced Techniques for Using Runes in Go Programming
Delve into the world of Go programming as we explore advanced techniques for handling runes. This blog provides practical code examples to master the use of runes in your Go projects.
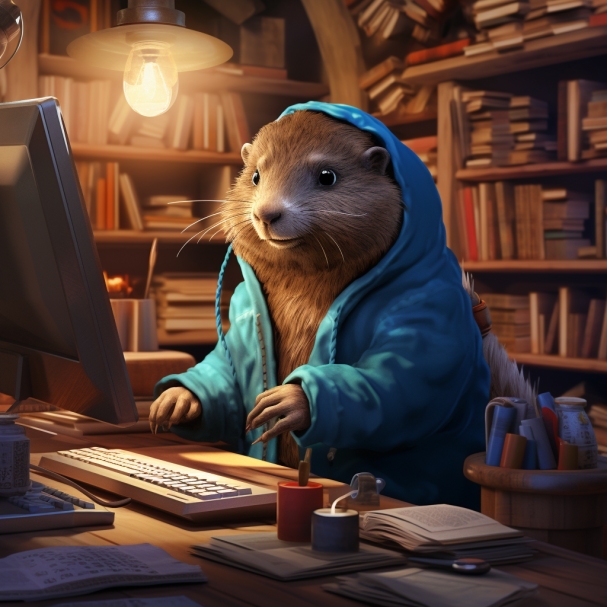
Unpacking the Functional Options Pattern in Go: Simplifying Configuration Complexity
The functional options pattern in Go provides a scalable and clean way to configure objects without overwhelming function signatures. Learn how to implement it with a practical example.
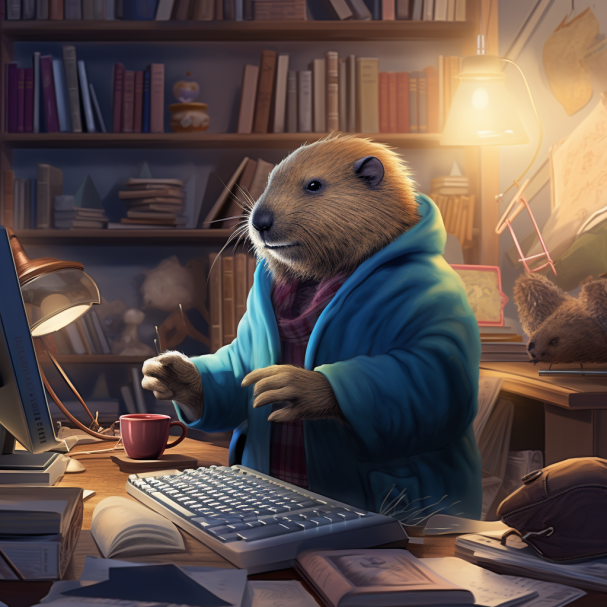
Unraveling the Mysteries of Go Struct Tags: A Comprehensive Guide
Ever wondered what struct tags in Go are all about? This post takes you through the what, why, and how of Go struct tags, including a handy list of tags and their specific uses. Perfect for both beginners and seasoned Go developers!
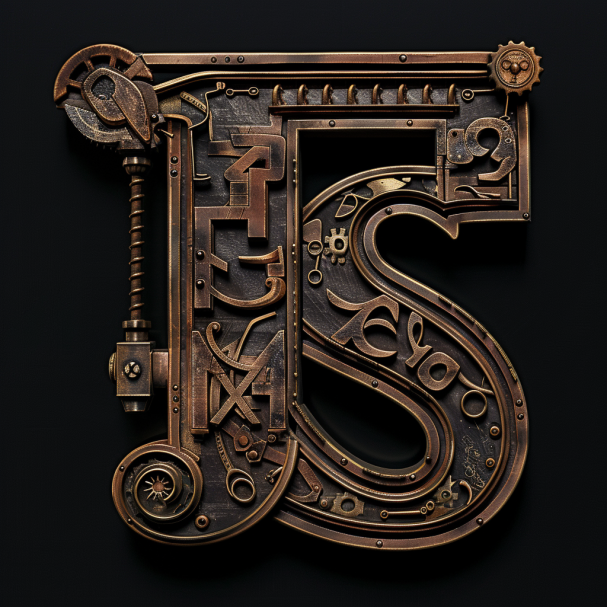
Yoda Conditions: Understanding Their Significance in Programming
In the vast expanse of programming best practices, there lies a curious, somewhat whimsical concept known as "Yoda conditions." This term, inspired by the iconic character Yoda from the "Star Wars" franchise, refers to a specific way of writing conditional statements in programming languages. The peculiarity of Yoda's speech, where he often places the object before the subject (e.g., "Ready are you?" instead of "Are you ready?"), mirrors the structure of these conditions. But what exactly are Yoda conditions, and why do they matter in the realm of coding?

Unveiling Programming's Revolutionary Feature: Neurolink Web Application Development
In a groundbreaking announcement that has set the developer community abuzz, the community has introduced an avant-garde feature that's set to redefine the paradigms of web application development: the Neurolink integration.
But wait, there's a twist that perfectly aligns with the spirits of April Fool's Day. This Neurolink isn't your run-of-the-mill brain-computer interface; instead, it harbors an innovative peer programming and code review functionality that ensures your development journey is as infinite as the loops it critiques.
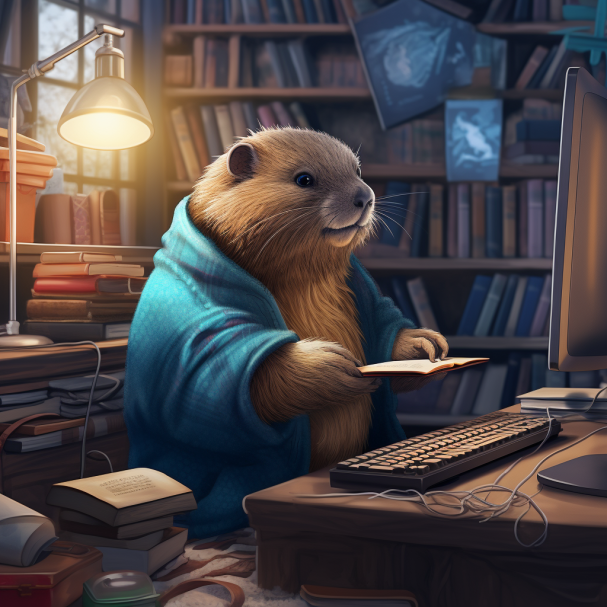
Understanding Pointers and uintptr in Go: Key Differences Explained
In the world of Go, pointers and uintptr are fundamental concepts that often cause confusion among newcomers. Both are used to deal with memory addresses, but they serve different purposes and obey different rules.
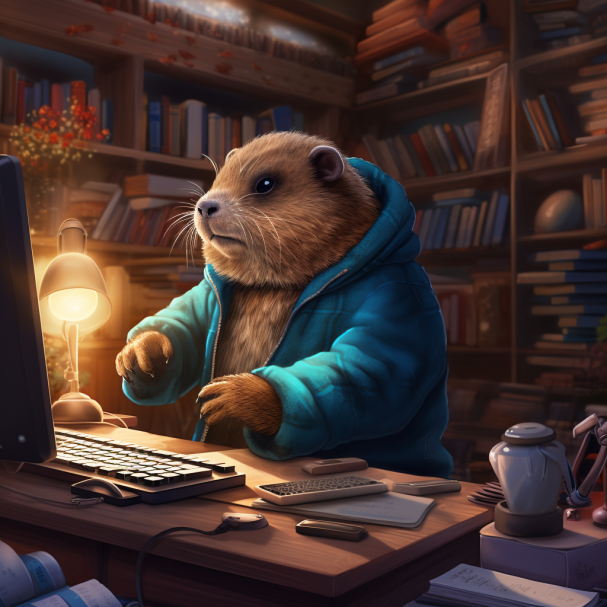
Understanding the Difference: make() vs new() in Go
In the world of Go programming, understanding the distinction between make() and new() functions is crucial for effective memory management and efficient coding practices. While both functions play pivotal roles in memory allocation, they serve different purposes and are used in different contexts.
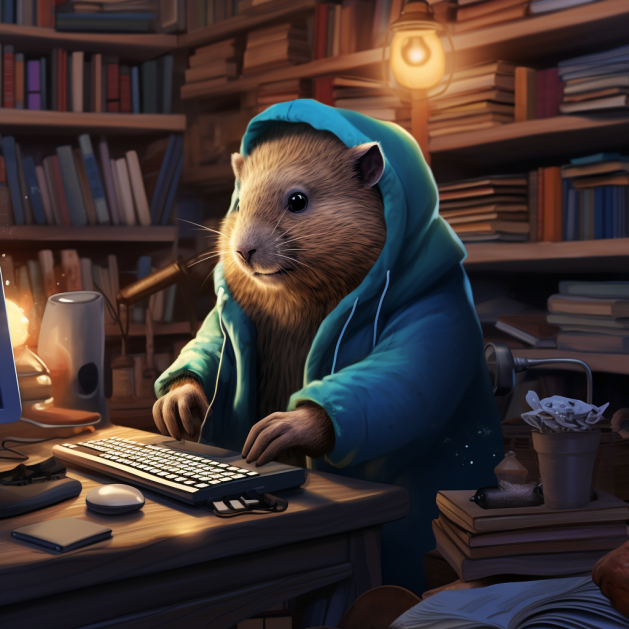
Understanding Iteration: Range vs. Index in GoLang
In GoLang, iteration over data structures is a fundamental concept that allows developers to traverse through arrays, slices, maps, and more. Two primary methods for iteration are using the range form and the traditional index-based iteration. Both approaches have their use cases, advantages, and limitations. Let's delve into these methods, understand their differences, and see when to use one over the other, with code examples to illustrate these concepts.
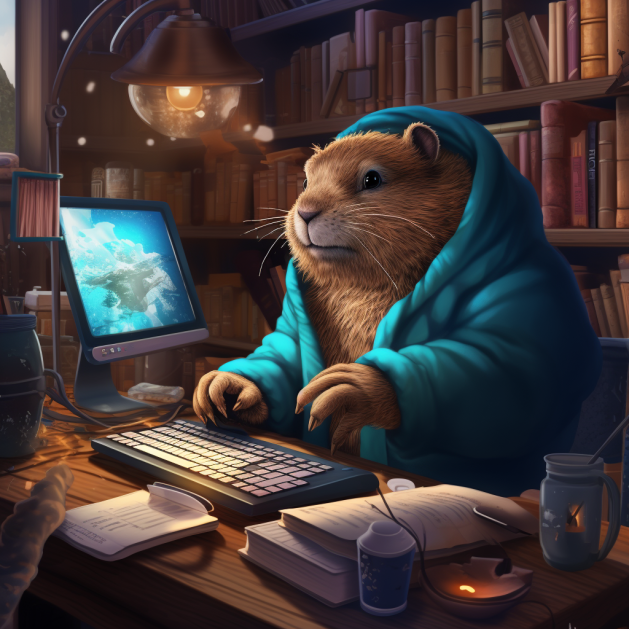
Exploring Array Techniques in Go: A Comprehensive Guide
Arrays are fundamental to any programming language, and Go is no exception. They offer a way to store and manipulate collections of data efficiently. In Go, arrays are value types, which means they are copied when assigned to a new variable or passed to functions. However, Go also provides slices, a more flexible and powerful alternative to arrays.

Understanding the Difference Between make and new in Go
In the Go programming language, memory allocation and initialization are crucial concepts that allow developers to manage data structures efficiently. Two built-in functions that play a significant role in this context are make and new. Although they might seem similar at first glance, they serve different purposes and are used in different scenarios.
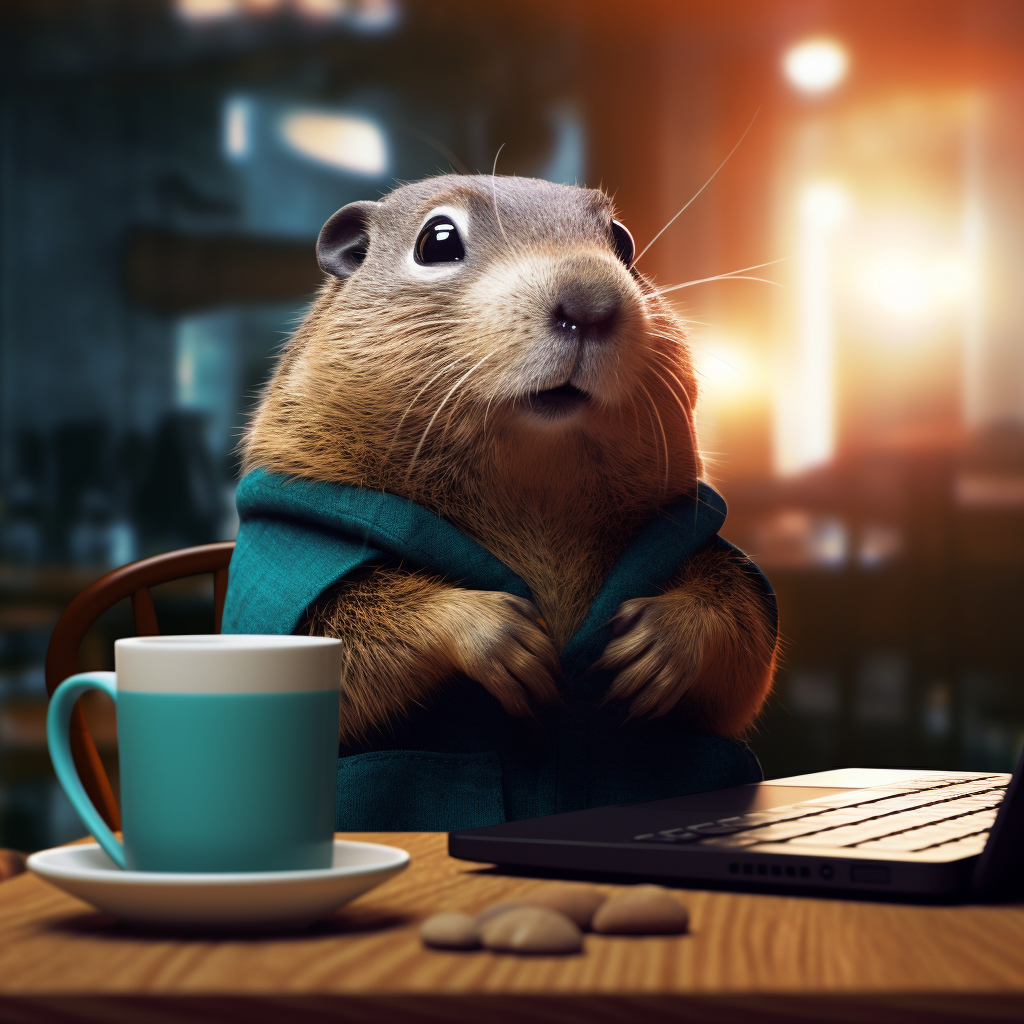
Understanding Type Aliases in Go: A Comprehensive Guide
Go is a statically typed programming language that has gained popularity for its simplicity, efficiency, and strong support for concurrent programming. One of the features that contribute to its simplicity and flexibility is the concept of type aliases.

Understanding Variable Passing into Go Routines
Go is a statically typed, compiled programming language designed for simplicity and efficiency, with a particular emphasis on concurrent programming. One of the core features of Go's concurrency model is goroutines, which are functions capable of running concurrently with other functions. A common question among Go developers, especially those new to the language, is why and how to pass variables into a goroutine.